Passing Data From Child to Parent in React
Let’s say you have a component Parent
that renders a Child
component. And, you want to pass some data from the Child
to Parent
component.
import React from 'react';
export function Child() {
return <p>Child</p>;
}
export default function Parent() {
return (
<>
<p>Parent</p>
<Child />
</>
);
}
Here’s how to pass data from a child component to a parent component in React:
- Create a function in the parent component that accepts a parameter
- Pass the function to the child component via props
- Call the function from the child and pass the data as argument
You can now access the data through the parameter in the parent function.
And if you are a visual learner, this is a diagram of how to enable child to parent communication in React:
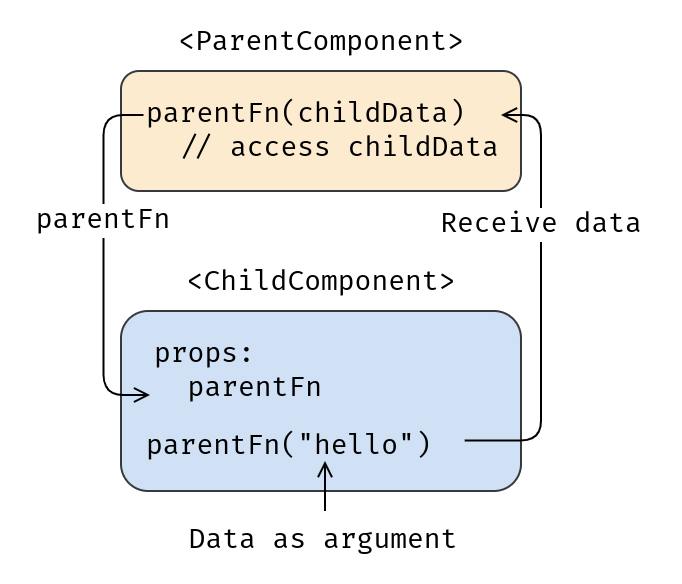
Child to Parent Data Pass
Here’s how the code from above should be modified to pass data from a child to parent component.
import React from 'react';
export function Child({ parentFn }) {
parentFn('hello, parent');
return <p>Child</p>;
}
export default function Parent() {
function parentFn(childData) {
console.log('Data from child:', childData); // hello, parent
}
return (
<>
<p>Parent</p>
<Child parentFn={parentFn} />
</>
);
}
From here you can further adjust the code to your needs. The parentFn
could be called from anywhere in Child
, for example, as an onClick
handler on a button.
function Child({ emitClickEvent }) {
function handleClick(event) {
emitClickEvent(event, 'Hello from child');
}
return (
<>
<p>Child</p>
<button onClick={handleClick}>Click me</button>
</>
);
}
export default function Parent() {
function handleClickEvent(event, data) {
// click event object, 'Hello from child'
console.log(event, data);
}
return (
<>
<p>Parent</p>
<Child emitClickEvent={handleClickEvent} />
</>
);
}
Here you can see that:
- You can pass the click event details from child to the parent
- You can use multiple arguments to pass different kinds of data